Postbacks
Learn about Lootably Postback Requests and how to configure them
What is a Postback?
A Postback URL is an HTTPS endpoint that receives a GET request when a user completes an offer or goal. Before sending each request, we adjust the request query parameters to include any relevant information you choose to receive. Configuring your postback is necessary to allow you to credit your users and receive other transaction information in real time.
Configuring your Postback
Begin by navigating to the postback tab on your placements dashboard.
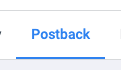
Below is a list of parameters you can use to configure your Postback URL.
As a reminder, all the parameters are sent as query parameters, which means they might need to be parsed into the proper types when your system receives the HTTPS request. The provided types below will help parse these parameters internally.
Parameter | Type | Description |
---|---|---|
{userID} | String | The ID of the user that completed the offer |
{transactionID} | String | The ID of this conversion in our database |
{ip} | String | The IP Address of the user when they started the offer |
{offerName} | String | The name of the offer the user completed |
{offerID} | String | The ID of the offer the user completed |
{numericOfferID} | Number | The ID of the offer the user completed stripped of any non-numeric characters. NOTE: This should only be used for systems where the database primary key is the offerID and must be a number. Most people should use {offerID} instead. |
{multistepOfferPercentageComplete} | Number | If the offer has multiple goals, this will return the percentage from 0-100 including decimals of how many goals the user has completed. If the completed offer does not contain any goals, this value will be empty |
{goalName} | String | The name of the goal the user completed. If the completed offer does not contain any goals, this value will be empty |
{goalID} | String | The ID of the goal the user completed. If the completed offer does not contain any goals, this value will be empty |
{revenue} | Number | The USD value you received from the completed offer or goal |
{currencyReward} | Number | The user's reward, calculated based on the settings from your placement's Currency tab |
{status} | "1" or "0" | The status of the offer, "1" reflects a completed conversion, while "0" reflects a chargeback. NOTE: Today, postbacks are only sent with a "1" status. If this changes in the future, we will be sure to provide ample notice to all publishers. |
{sid2} | String | An additional custom parameter you can pass through to the offerwall or API. If the offer is not supported, this value will be empty. You can set this by adding an sid2 parameter on either the offerwall link or the tracking url. |
{sid3} | String | An additional custom parameter you can pass through to the offerwall or API. If the offer is not supported, this value will be empty. You can set this by adding an sid3 parameter on either the offerwall link or the tracking url. |
{sid4} | String | An additional custom parameter you can pass through to the offerwall or API. If the offer is not supported, this value will be empty. You can set this by adding an sid4 parameter on either the offerwall link or the tracking url. |
{sid5} | String | An additional custom parameter you can pass through to the offerwall or API. If the offer is not supported, this value will be empty. You can set this by adding an sid5 parameter on either the offerwall link or the tracking url. |
{hash} | String | The SHA256 hash for the postback, which is used to independently verify the postback was sent from Lootably. Read more below. |
Here is an example of what the postback URL might look like. Your postback URL can include all, any, or none of the above parameters.
https://api.example.com/postback/lootably?userID={userID}&reward={currencyReward}&revenue={revenue}&offerName={offerName}&offerID={offerID}&userIP={ip}&hash={hash}
Response
When the postback request has been successfully processed by your system, you must return a "1" response body. If your system encounters an error while processing the postback request, you can return an error message.
Our system only accepts a "1" response body as an indicator of a successful postback request. Any other value will be treated as a failure by our system.
Additionally, you can configure "Postback Retries." Postback Retries is a number between 0-5, and we will attempt to retry the postback request until either your server responds with a "1" response body or we run out of retry attempts.
By default, postbacks are NOT resent unless you explicitly set a Postback Retries value.
Validating Postbacks
To validate that a postback originated from Lootably's system:
- Concatenate userID, ip, revenue, currencyReward, and your Postback Secret string from your placement's Postback settings page.
- Generate a SHA256 hash of the concatenated string
- Compare your generated SHA256 hash to the value of the
{hash}
postback parameter.- If the hashes match, you know the postback originated from our system
Example hashing logic implementations:
sha256(userID + ip + revenue + currencyReward + "YOUR_POSTBACK_SECRET")
hash("sha256", userID . ip . revenue . currencyReward . "YOUR_POSTBACK_SECRET")
Node Module
We provide a plug-and-play node module that performs the hash generation for you.
View the full documentation on NPM
Installation
npm i lootably-postback-hash
Usage
import { generateSHA256PostbackHash } from 'lootably-postback-hash';
const hash = generateSHA256PostbackHash({
userID: 'example_user_123',
ip: '192.0.2.1',
revenue: '0.5000',
currencyReward: '100.00',
postbackSecret: process.env.LOOTABLY_POSTBACK_SECRET,
});
console.log('Generated hash:', hash);
Updated 16 days ago